Service vs Repository: What Should You Use Inside?
May 11, 2025
Basic Structure
Before we dive in, let’s briefly look at how we typically structure a Backend Project.
Most basic and widely used structure is MVC Pattern, which consists of Controller - Service - Repository.
- Controller handles request and response.
- Service contains Business logic and implements core funcionality.
- Repository hanles database interations.
Then, what should we do if one service needs to use the logic from another service?
Service
First, here’s an example of how a service can call another service.
java@Service @RequiredArgsConstructor @Transactional(readOnly = true) public class AttendeeService { private final AttendeeRepository attendeeRepository; private final LineService lineService; }
Advantage
- By reducing code duplication, we improve maintainability.
- Business logic can be managed in a single place.
Disadvantage
- It increases complexity by introducing dependencies from the other service.
- It can lead to errors caused by cyclic dependencies.
- If another service calls it, a cascading test might be required.
Repository
Here’s an example of how a service can call a repository.
java@Service @RequiredArgsConstructor @Transactional(readOnly = true) public class AttendeeService { private final AttendeeRepository attendeeRepository; private final LinRepository lineRepository; }
Advantage
- It helps maintain a consistent structure when a service directly calls its repository.
- It improves testability by removing unnecessary dependencies on other services.
Disadvantage
- Code duplication can hurt maintainability.
- However, this can be mitigated by extracting common logic into utility classes.
Result
Before finalizing the structure, I searched through various blogs and asked AI for advice.
But I was still confused about how to structure the services, so I asked in the Threads app and gathered opinions through a poll.
As a result, conclusion is services should only communicate with repositories.
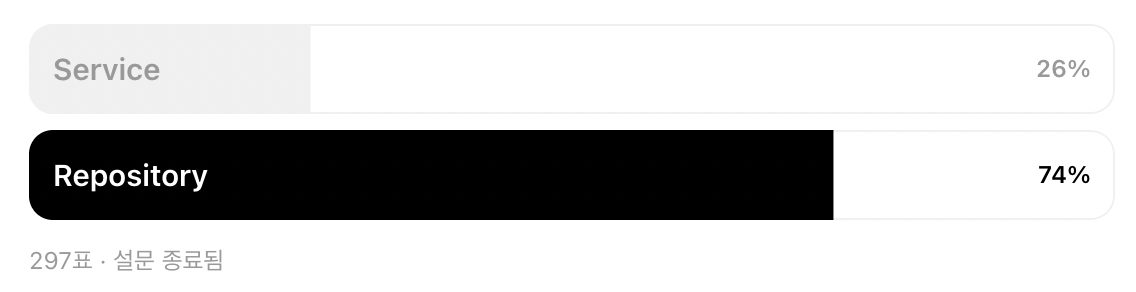
As shown in the image above, we can see that Service is the most selected option.
One person also left a comment, as shown below.
Consistency is very important.
If one service calls another service, and other service only calls a repository, it can make maintainability difficult.
If we maintain consistency, code duplication won’t be a big problem.
I agree with the comment above, and I believe that the Service → Repository structure is the way to go.
As a developer, I’ll avoid using things without proper consideration and always aim to think critically and provide clear reasoning.
Share this post
Comments (0)
No comments yet. Be the first to comment!